How to start programming
- Alice Eleanor Matthews
- Jun 1, 2020
- 9 min read
Updated: Jun 8, 2020

Hey guys! I hope this is a little helpful for you getting started. Please note, I am also a beginner and I have mostly self-taught myself 'everything I know' (which isn't much). But because of the nature of how I learned, I hope I can help you guys feel a little less stuck and confused on how to start! Being a student of physics at Manchester, they really throw you in the deep end to figure it all out, so I just spelt out a few things I wish I knew before I started.
Firstly, I just want to quickly say, if you feel you really want to start programming, but are worried about a few things before reading this post on how to start, I really recommend reading my post 'Programming Myths: DEBUNKED'.
Find out what language you should learn
Ask yourself: why do you want to code?
Do you want to build complex data structures, perform data-analysis, run algorithms, perform memory allocation? Mostly for science, gaming and data analysis I assume.. Then you'll need something like C++ or Python. Or are you more interested in website development? Then you’ll most likely need html and CSS (html for the ‘objects’ and CSS for the ‘styling’). In the future, you might need to add more complexity to your website and for example. you might need to add in payment systems, databases. For this type of functionality, you will need PHP, SQL and JavaScript. Or do you want to make video games? In that case, Unity and C will be needed. For App design, you will need to think about whether or not you want it to be compatible across multiple operating systems, such as Android or iOS. This will determine if you need C, swift or Java for example. There is an abundance of information online to help you figure out what type of language you should start with, but I hope this explains that basically, for different projects and types of code you want to write, there is a language specifically for it and you need to think carefully about what one you need.
Brain hurting yet?...
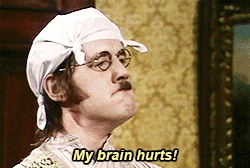
If you're still not sure about a certain project and just want to start programming, I would recommend starting with either Python, Java and C++, as these are very popular and good starting points.
C is the great grandmother of most languages. Learning C (or C++) will teach you how to think like a computer, as it forces you to think about computing technicalities like memory allocation and structure of code. It teaches you how to program at the lowest ‘hardware’ level (C is the foundation for Linux). It will force you to debug your code, as errors aren't pointed out so easily as in higher level languages such as Python, and this is an important skill to have, as it teaches you to figure out why you had an error and to fix it yourself.
It also depends if you want to go into the deep end, for example, learn C++ and higher level languages will seem like a walk in the park. Alternatively, you could start with a higher level language first and build up to (or bog down to - I should say), the more complex languages like C++. Learning a C language might seem like a waste of time if you just want to ‘make stuff’ and make use of programming, and to force yourself to learn the complexity of things like memory allocation and data structures, might cause a lot of unnecessary pain and trogging through code (with some confusion too probably). So if you want to see results straight away, it might be worth starting with a higher level language, such as Python. If you’re a physicist, I would recommend starting with Python and C++. If you want to build websites, start with HTML and CSS (these go hand in hand) and you will most likely find that when you need to, you can implement bits of PHP and JavaScript into your website at a later date when you are more confident.
You might also want to take other shortcuts, for example, learn a language that uses important concepts like Object Oriented Programming (OOP). For example Python, Java or C++ : once you have learnt the OOP concepts, they can be used for other programming languages like Java and Ruby, etc. The concepts are the same, just the syntax is different. Python is also an OOP language, and the syntax is a lot cleaner, for example:, if I wrote two programs in both C++ and Python to ask my name and print a statement, it might look something like this:
C++ code to output "Hey, Alice"
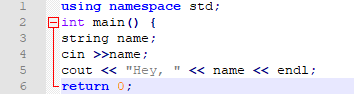
uses 6 lines of code to get the same output whereas Python would only need 2 lines of code:
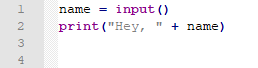
Here is a really great blog post comparing C++ and Python if you are unsure of the differences.
Useful Resources to find the right language for you!
Here are some useful posts which I used as a reference here, and are really helpful with deciding what language you could start with:
To help you decide, think about what you want to be doing, write it down in a mind map and get yourself organised. Once you have a clear idea on your project, it will be easier to recognise what sort of program and language you will need to use. Programming is like learning an actual language, if you pick up one, it becomes easier to pick up others (apparently..).. I do believe this is true, but, not relating to the syntax or 'language' (as that varies a lot) but with the problem-solving, analytical and logical thinking abilities you pick up by learning to code. You will take these skills with you on to any new project, be in a programming one or not - and these are great skills to learn!
"Learning to Code teaches you how to think"
Download editing software
Where the hell do I write my code and how do I actually run it?
This might be obvious to some people but I didn't want to skip any steps, and this is something I definitely didn’t know when I started.
See this website for an example of how to put your code in, and run it, to see the output - here you can choose the language you want to run.
The reason I show this is because I wanted it to be an easy first example of what ‘running code’ looks like. You type in ‘somewhere’, i.e. in a terminal or editing software, and then you run it. There are also additional stages such as ‘compiling’ code before it is actually run and this can be confusing. But basically, some 'compiled' languages do some stuff before they run the program. When you press 'run', the program will compile, which basically puts all the 'code' into an executable file, of which is then 'run' after this step is completed. Interpreted languages, however, run an executable during 'run time' so this makes them a little slower - See here for a really good description of what compiled and interpreted languages mean.
So how do we run code?
There are different ways to run your code.
1. Directly from a terminal/command line
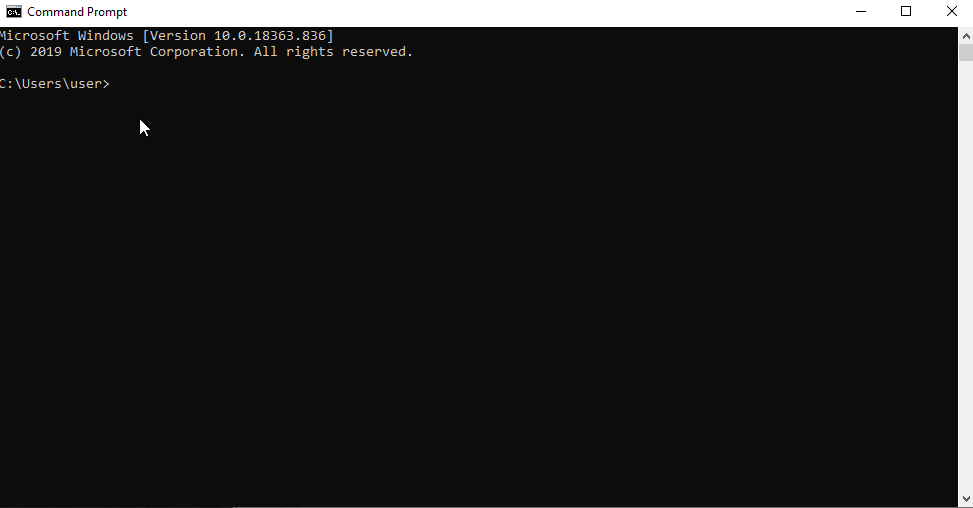
Windows: Press the windows key and type in ‘cmd’ and press enter
Mac: Press the Spotlight icon in the upper-right corner of the menu bar, or press Command-Space bar and type in ‘terminal’ and press enter.
Although, I only really recommend using cmd when you are using a Linux machine. It's the only place where it is actually easier to run from the terminal than it is a script editor (Speaking from Windows experience only, Mac I am not sure).
To run from the terminal, you need to be in the correct ‘working directory’ of the program you want to use. You can navigate to this using the command prompt 'cd' and then the path. Here are some shortcuts on using the command prompt.
You will then need to type in the file name from your terminal and run the program. For example, if I had a program called "Alices_Code.py" (.py for Python) then I would type in the terminal
> py Alices_Code.py
The py before the name of my file tells the prompt that I want to run a python script.
See here on how to use the command line to run Python scripts. This stuff is more complicated than using a user friendly interface such as a script editor, but I wanted to mention it in case you ever wondered what people are doing in that curious little black box. Also all of this stuff is google-able so don't feel scared to try it out!
2. Using a Script Editor
Visual Studio Code (VSC):
See this post for more examples of other script editors out there, but I have only recommended ones I have used myself or friends have recommended.
A few tips when using a script editor (such as VSC)
You can download pretty themes!
Save your files correctly, using good, descriptive naming conventions (even add on the end of the date e.g. "my_file_01052020" to ensure you don't overwrite files when editing versions, and also lose them because of names like "my_file_NEWEST" , "my_file_TO_SUBMIT" or "my_file_FINAL", as you can see, when you come to submit this to the assessment board, which bloody one do you pick?!
if you already have a project and are unsure on how to find it on VSC, go to your file explorer and open it from there. (find the file of code you want to edit and right click and open in VSC). VSC should automatically open the last file you were editing.
Useful resources to get started
1. Online Courses
2. YouTube
and many many more! Please make use of YouTube and always refer back to it if you get stuck because there is honestly so much on there!
3. Books
Books are a really nice way to learn to code without the distractions of the internet (which sometimes lead to horrible rabbit holes of code that are far too advanced for the tool I need and can waste a lot of time). A book goes at a nice pace and takes you through the concepts at a good rate. I used a C++ book in my third year OOP project and it was really helpful! Here are a couple of resources I have linked below anyway but again, there are loads of recommended books online. With the books, I would say don't go crazy and get a 'Machine Learning Data Analysis and Artificial Intelligence for Python" book... I would start with basic concepts and build up. I also wouldn't recommend anything that says "Learn Python in one day" - it just seems unrealistic to me and probably not the best book for a 'to-be' long term programmer.
What to do when you’re stuck
Firstly, it's OK to get stuck, even on the most basic things. Everyone does it. Trust me. The first thing you should do is try and solve the problem yourself first. Don't get upset or angry like moss.
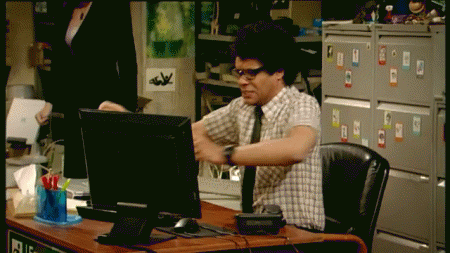
I always recommend that when you have a problem, it's time for a tea break. Fresh eyes always help solve issues. Especially with code (one becomes blind to reality when being in the matrix for so long)! You'll come back with a new wave of energy and calm mind, ready to fight and figure out what the issue is..
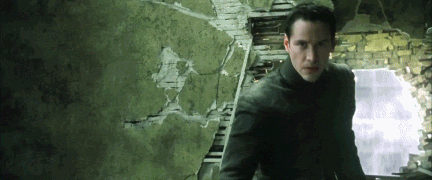
1. Solve the Problem Yourself
It depends on the problem, but for example, if it is a basic syntax error, then it is most likely a missing bracket, something has been left open '{' but no '}' for example.. or an indentation error, or something silly like misnaming a variable and using the wrong one throughout. So first: Check the error message, which might point to the line of code with the problem, and then check your code. (look at the lines before where the error is pointed as it might be an open bracket too).
If it is a deeper issue, maybe your program isn't working how you expected, then you will need to put in some more effort here, and dissect what the real issue is. I would go through your code step by step (line by line) and check your logic, to ensure it is doing what you really want it to do.
2. Ask the internet or look it up in a book.
Secondly, if you are still unsure, google it. Copy the error message into google. I can guarantee you as a beginner, someone has had the same problem as you before, and there is a solution on the internet somewhere. Type in your error code on google with "quotation marks" around it to ensure the whole phrase is searched, not just the individual terms and see what the internet has to say. Alternatively, use your books to look up in the index some of the words you might be using in your code or that came up in the error message.
Where do I look?
Chats/Forums
Google it (seriously)
Common helpful websites are:
GitHub Community Forum (also if you’re starting to code, get a GitHub account)
StackOverflow (which I like to refer to as ‘Stuck’ overflow)
3. Come back to it the next day and repeat steps one and two.
Just remember, learning to code teaches you how to think logically, analytically and how to solve problems. Getting things wrong and figuring them out is how you learn these skills. So try not to get worked up and bogged down in errors. Take it as an opportunity to learn and grow, and you'll get there in the end! There is no greater feeling that fixing something that has been long broken! You will feel invisible!
Best of luck learning guys and if you're feeling stuck just keep at it because nothing worth doing is ever easy and you will get there if you persevere!
I hope this was helpful, it can be really difficult to get started and if there is something I haven't covered or you would like to know more about please leave a comment on this post!
Comments